El objetivo de esta entrada es proporcionar los números Egipcios del uno al cien:
Te proporciono otra serie de números Egipcios pero esta vez, de cien en cien hasta el mil:
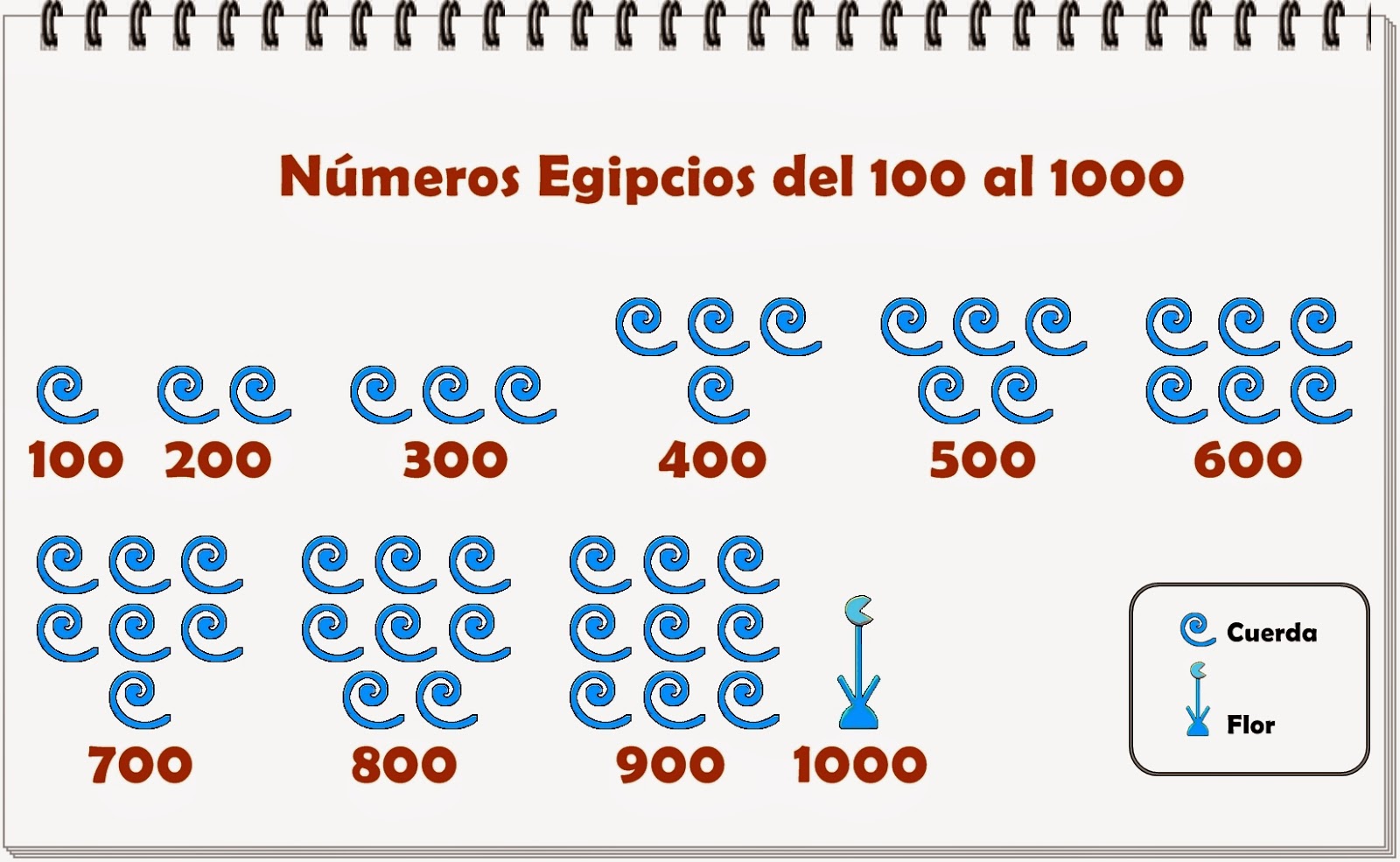
Espero y te sirvan...Saludos!!
package quicksort; //@author Oliver //http://blog2000.blogspot.mx/ import java.awt.*; import java.awt.event.*; import javax.swing.*; public class Quicksort extends JFrame implements ActionListener{ JLabel etiqueta1, metodos; JTextField numero; JButton boton0, boton1, boton3, salir; int limite; public Quicksort(){ // Titulo de pestaña de ventana super("Quicksort Recursivo"); Container contenedor=getContentPane(); contenedor.setLayout(new FlowLayout()); inicializar(); // Titulo principal etiqueta1=new JLabel("QUICKSORT RECURSIVO"); etiqueta1.setBackground(Color.BLACK); // Color de fondo etiqueta1.setForeground(Color.BLACK); // Color de texto etiqueta1.setFont(new Font("Consolas", 0, 23)); // Tipo y tamaño de letra contenedor.add(etiqueta1); numero=new JTextField(15); contenedor.add(numero); // Boton para ingresar cadenas boton0=new JButton("Ingresa"); boton0.addActionListener(this); boton0.setBackground(Color.WHITE); // Color de fondo boton0.setForeground(Color.BLUE); // Color de texto boton0.setFont(new Font("Consolas", 0, 20)); // Tipo y tamaño de letra contenedor.add(boton0); // Boton para mostrar cadenas boton3=new JButton("Muestra"); boton3.addActionListener(this); boton3.setBackground(Color.WHITE); // Color de fondo boton3.setForeground(Color.BLUE); // Color de texto boton3.setFont(new Font("Consolas", 0, 20)); // Tipo y tamaño de letra contenedor.add(boton3); // Subtitulo Metodo metodos=new JLabel("MÉTODO n"); metodos.setBackground(Color.BLACK); // Color de fondo metodos.setForeground(Color.BLACK); // Color de texto metodos.setFont(new Font("Consolas", 0, 20)); // Tipo y tamaño de letra contenedor.add(metodos); // Boton Metodo Quicksort Recursivo boton1= new JButton("Método Quicksort R."); boton1.setBackground(Color.BLUE); // Color de fondo boton1.setForeground(Color.WHITE); // Color de texto boton1.setFont(new Font("Consolas", 0, 20)); // Tipo y tamaño de letra contenedor.add(boton1); boton1.addActionListener(this); // JButton que agrega un boton Salir salir=new JButton("Salir"); salir.setBackground(Color.WHITE); salir.setForeground(Color.BLUE); salir.setFont(new Font("Consolas", 0, 20)); // Tipo y tamaño de letra contenedor.add(salir); salir.addActionListener(this); } // Main Principal public static void main(String[] args) { Quicksort aplicacion= new Quicksort(); // Tamaño de ventana aplicacion.setSize(300,300); // Visible aplicacion.setVisible(true); // Centrada aplicacion.setLocationRelativeTo(null); // Cierre aplicacion.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } // Cantidad de valores a ordenar String a[]; int i=0; String salida="<html><p style="color: #000000; font-family: consolas; font-size: 15px;">Orden Original: n"; // Metodo para ingresar valores public void actionPerformed(ActionEvent evento){ if (evento.getSource()==boton0){ a[i]=numero.getText(); salida+=" "+a[i]; i++; numero.setText(""); if(i==a.length){ JOptionPane.showMessageDialog(null,"<html><p style="color: #000000; font-family: consolas; font-size: 15px;">Fin de Ingreso"); } } // Accion para salir if(evento.getSource().equals(salir)) { System.exit(0); } if(evento.getSource()==boton1){ quicksort_recursivo(a); } if (evento.getSource()==boton3){ salida+="n<html><p style="color: #000000; font-family: consolas; font-size: 15px;"> Nuevo Orden: n"; muestra(a); } } public void inicializar(){ String texto = JOptionPane.showInputDialog("<html><p style="color: #000000; font-family: consolas; font-size: 15px;">¿Cuantos nombres deseas ingresar?"); limite = Integer.parseInt(texto); a = new String [ limite]; } // Metodo Quicksort Recursivo public void quicksort_recursivo(String a1[]) { reduce_recursivo(a1, 0, a1.length - 1); JOptionPane.showMessageDialog(null,"<html><p style="color: #000000; font-family: consolas; font-size: 15px;">Cadenas Ordenados"); } // Reduce public void reduce_recursivo(String a1[], int ini, int fin) { int izq = ini, der = fin, pos = ini; String aux=""; boolean band = true; while (band) { band = false; while ((a1[der].charAt(0) >= a1[pos].charAt(0)) && (pos != der)) der = der - 1; if (pos != der) { aux = a1[pos]; a1[pos] = a1[der]; a1[der] = aux; pos = der; while ((a1[izq].charAt(0) < a1[pos].charAt(0)) && (pos != izq)) izq = izq + 1; if (pos != izq) { band = true; aux = a1[pos]; a1[pos] = a1[izq]; a1[izq] = aux; pos = izq; } } } if (ini < (pos - 1)) { reduce_recursivo(a1, ini, pos - 1); } if ((pos + 1) < fin) { reduce_recursivo(a1, pos + 1, fin); } } // Metodo para mostrar valores public void muestra(String a2[]){ for (int i=0;i<a2.length;i++){ salida+=a2[i]+" "; } JOptionPane.showMessageDialog(null,salida); } }
package busquedabinaria; //@author Oliver //http://blog2000.blogspot.mx/ import java.awt.*; import java.awt.event.*; import javax.swing.*; public class BusquedaBinaria extends JFrame implements ActionListener{ JLabel etiqueta1, metodos; JTextField numero; JButton boton0, boton1, boton2, salir; int limite; public BusquedaBinaria(){ // Titulo de pestaña de ventana super("Búsqueda Binaria"); Container contenedor=getContentPane(); contenedor.setLayout(new FlowLayout()); inicializar(); // Titulo principal etiqueta1=new JLabel("BÚSQUEDA BINARIA"); etiqueta1.setBackground(Color.BLACK); // Color de fondo etiqueta1.setForeground(Color.BLACK); // Color de texto etiqueta1.setFont(new Font("Consolas", 0, 23)); // Tipo y tamaño de letra contenedor.add(etiqueta1); numero=new JTextField(15); contenedor.add(numero); // Boton para ingresar cadenas boton0=new JButton("Ingresa Nombre del Alumno"); boton0.addActionListener(this); boton0.setBackground(Color.WHITE); // Color de fondo boton0.setForeground(Color.BLUE); // Color de texto boton0.setFont(new Font("Consolas", 0, 20)); // Tipo y tamaño de letra contenedor.add(boton0); // Subtitulo Metodo metodos=new JLabel("MÉTODO n"); metodos.setBackground(Color.BLACK); // Color de fondo metodos.setForeground(Color.BLACK); // Color de texto metodos.setFont(new Font("Consolas", 0, 20)); // Tipo y tamaño de letra contenedor.add(metodos); // Boton Metodo Busqueda Binaria boton2= new JButton("Método Búsqueda Binaria"); boton2.setBackground(Color.BLUE); // Color de fondo boton2.setForeground(Color.WHITE); // Color de texto boton2.setFont(new Font("Consolas", 0, 20)); // Tipo y tamaño de letra contenedor.add(boton2); boton2.addActionListener(this); // JButton que agrega un boton Salir salir=new JButton("Salir"); salir.setBackground(Color.WHITE); salir.setForeground(Color.BLUE); salir.setFont(new Font("Consolas", 0, 20)); // Tipo y tamaño de letra contenedor.add(salir); salir.addActionListener(this); } // Main Principal public static void main(String[] args) { BusquedaBinaria aplicacion= new BusquedaBinaria(); // Tamaño de ventana aplicacion.setSize(300,300); // Visible aplicacion.setVisible(true); // Centrada aplicacion.setLocationRelativeTo(null); // Cierre aplicacion.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } // Cantidad de valores a ordenar String a[]; int i=0; String salida="<html><p style="color: #000000; font-family: consolas; font-size: 15px;">Orden Original: n"; // Metodo para ingresar valores public void actionPerformed(ActionEvent evento){ if (evento.getSource()==boton0){ a[i]=numero.getText(); salida+=" "+ a[i]; i++; numero.setText(""); if(i==a.length){ JOptionPane.showMessageDialog(null,"<html><p style="color: #000000; font-family: consolas; font-size: 15px;">Fin de Ingreso"); } } // Accion para salir if(evento.getSource().equals(salir)) { System.exit(0); } if(evento.getSource()==boton2){ String dato; dato = JOptionPane.showInputDialog(null,"<html><p style="color: #000000; font-family: consolas; font-size: 15px;">¿Que alumno quieres buscar?"); Binaria(a, dato); } } public void inicializar(){ String texto = JOptionPane.showInputDialog("<html><p style="color: #000000; font-family: consolas; font-size: 15px;">¿Cuantos alumnos deseas ingresar?"); limite = Integer.parseInt(texto); a = new String [limite]; } // Metodo Busqueda Binaria public void Binaria(String A[], String X) { int izq, der, cen = 0; boolean band=false; izq=0; der=A.length-1; while((izq<=der) && (band==false)) { cen=((izq+der)/2); if((int)X.charAt(0) == (int)A[cen].charAt(0)){ band=true; } else { if((int)X.charAt(0)<=(int)A[cen].charAt(0)){ izq=cen+1; } else der=cen-1; } } if(band==true){ JOptionPane.showMessageDialog(null,"<html><p style="color: #000000; font-family: consolas; font-size: 15px;">El alumno se encuentra en la posicion:"+cen); } else{ JOptionPane.showMessageDialog(null,"<html><p style="color: #000000; font-family: consolas; font-size: 15px;">El alumno no se encuentra..."); } } // Metodo para mostrar valores public void muestra(String a2[]){ for (int i=0;i<a2.length;i++){ salida+=a2[i]+" "; } JOptionPane.showMessageDialog(null,salida); } }
package libreria; //@author Oliver //http://blog2000.blogspot.mx/ import java.io.*; import java.awt.*; import javax.swing.*; import java.awt.event.*; import java.util.Scanner; // Clase PRINCIPAL public class Libreria extends JFrame implements ActionListener { private JMenuBar barra; private JMenu menu1, menu2, menu3; private JMenuItem submenu1, submenu2, submenu3; private JLabel bienvenido; private JLabel lbl1, lbl2, lbl3, lbl4; private JTextField tituloL, edicion, precio, clave; private JButton guardar, mostrar, limpiar, salir; public Libreria() { barra = new JMenuBar(); barra.setBounds(0,0,615,30); //(x, y, width, height) menu1 = new JMenu ("<html><p style="border-left: solid 10px #B40404; padding-left: 10px;">Archivo</p></html>"); menu1.setForeground(new Color(0xFFFFFF)); menu2 = new JMenu("<html><p style="border-left: solid 10px #B40404; padding-left: 10px;">Acciones</p></html>"); menu2.setForeground(new Color(0xFFFFFF)); menu3 = new JMenu("<html><p style="border-left: solid 10px #B40404; padding-left: 10px;">Edición</p></html>"); menu3.setForeground(new Color(0xFFFFFF)); barra.add(menu1); barra.add(menu2); barra.add(menu3); barra.setBackground(new Color(0x000000)); submenu1 = new JMenuItem("Salir"); submenu2 = new JMenuItem("Limpiar"); submenu3 = new JMenuItem("Mostrar"); menu1.add(submenu1); menu2.add(submenu2); menu2.add(submenu3); // JLabel que agrega un TITULO PRINCIPAL bienvenido = new JLabel("<html><p style="border-left: solid 10px #B40404; border-top: solid 10px #B40404; border-right: solid 10px #B40404; padding-left: 10px; padding-right: 10px;">LIBRERIA</p></html>"); bienvenido.setBounds(130, 30, 350, 100); // (x, y, width, height) bienvenido.setFont(new Font("ALGERIAN", 0, 50)); bienvenido.setForeground(new Color(0x000000)); // JLabel que agrega un TEXTO lbl1 = new JLabel("<html><p style="border-left: solid 10px #B40404; padding-left: 10px;">Titulo del libro:</p></html>"); lbl1.setBounds(30, 40, 500, 200); // (x, y, width, height) lbl1.setFont(new Font("Impact", 0, 30)); lbl1.setForeground(new Color(0x000000)); // JTextField que agrega un CAMPO DE TEXTO tituloL = new JTextField(""); tituloL.setBounds(255, 125, 275, 30); // (x, y, width, height) tituloL.setFont(new Font("Arial", 0, 20)); tituloL.setForeground(new Color(0x000000)); tituloL.setBackground(new Color(0xDDDDDD)); // JLabel que agrega un TEXTO lbl2 = new JLabel("<html><p style="border-left: solid 10px #B40404; padding-left: 10px;">Edicion:</p></html>"); lbl2.setBounds(30, 80, 500, 200); // (x, y, width, height) lbl2.setFont(new Font("Impact", 0, 30)); lbl2.setForeground(new Color(0x000000)); // JTextField que agrega un CAMPO DE TEXTO edicion = new JTextField(""); edicion.setBounds(165, 165, 365, 30); // (x, y, width, height) edicion.setFont(new Font("Arial", 0, 20)); edicion.setForeground(new Color(0x000000)); edicion.setBackground(new Color(0xDDDDDD)); // JLabel que agrega un TEXTO lbl3 = new JLabel("<html><p style="border-left: solid 10px #B40404; padding-left: 10px;">Precio:</p></html>"); lbl3.setBounds(30, 120, 500, 200); // (x, y, width, height) lbl3.setFont(new Font("Impact", 0, 30)); lbl3.setForeground(new Color(0x000000)); // JTextField que agrega un CAMPO DE TEXTO precio = new JTextField(""); precio.setBounds(155, 205, 375, 30); // (x, y, width, height) precio.setFont(new Font("Arial", 0, 20)); precio.setForeground(new Color(0x000000)); precio.setBackground(new Color(0xDDDDDD)); // JLabel que agrega un TEXTO lbl4 = new JLabel("<html><p style="border-left: solid 10px #B40404; padding-left: 10px;">Clave:</p></html>"); lbl4.setBounds(30, 160, 500, 200); // (x, y, width, height) lbl4.setFont(new Font("Impact", 0, 30)); lbl4.setForeground(new Color(0x000000)); // JTextField que agrega un CAMPO DE TEXTO clave = new JTextField(""); clave.setBounds(140, 245, 390, 30); // (x, y, width, height) clave.setFont(new Font("Arial", 0, 20)); clave.setForeground(new Color(0x000000)); clave.setBackground(new Color(0xDDDDDD)); // JButton que agrega un BOTON guardar = new JButton("<html><p style="border-left: solid 10px #B40404; padding-left: 10px;">Guardar</p></html>"); guardar.setBounds(30, 300, 130, 30); // (x, y, width, height) guardar.setFont(new Font("Arial", 0, 20)); guardar.setForeground(new Color(0xFFFFFF)); guardar.setBackground(new Color(0x000000)); guardar.setToolTipText("Pulsa para guardar"); // JButton que agrega un BOTON mostrar = new JButton("<html><p style="border-left: solid 10px #B40404; padding-left: 10px;">Mostrar</p></hmtl>"); mostrar.setBounds(170, 300, 130, 30); // (x, y, width, height) mostrar.setFont(new Font("Arial", 0, 20)); mostrar.setForeground(new Color(0xFFFFFF)); mostrar.setBackground(new Color(0x000000)); mostrar.setToolTipText("Pulsa para mostrar"); // JButton que agrega un BOTON limpiar = new JButton("<html><p style="border-left: solid 10px #B40404; padding-left: 10px;">Limpiar</p></hmtl>"); limpiar.setBounds(310, 300, 130, 30); // (x, y, width, height) limpiar.setFont(new Font("Arial", 0, 20)); limpiar.setForeground(new Color(0xFFFFFF)); limpiar.setBackground(new Color(0x000000)); limpiar.setToolTipText("Pulsa para limpiar"); // JButton que agrega un BOTON salir = new JButton("<html><p style="border-left: solid 10px #B40404; padding-left: 10px;">Salir</p></html>"); salir.setBounds(450, 300, 130, 30); // (x, y, width, height) salir.setFont(new Font("Arial", 0, 20)); salir.setForeground(new Color(0xFFFFFF)); salir.setBackground(new Color(0x000000)); salir.setToolTipText("Pulsa para salir"); guardar.addActionListener(this); mostrar.addActionListener(this); limpiar.addActionListener(this); salir.addActionListener(this); submenu1.addActionListener(this); submenu2.addActionListener(this); submenu3.addActionListener(this); getContentPane().add(guardar); getContentPane().add(mostrar); getContentPane().add(limpiar); getContentPane().add(salir); getContentPane().add(lbl1); getContentPane().add(lbl2); getContentPane().add(lbl3); getContentPane().add(lbl4); getContentPane().add(bienvenido); getContentPane().add(tituloL); getContentPane().add(edicion); getContentPane().add(precio); getContentPane().add(clave); getContentPane().add(barra); add(Imagen1()); //Ventana GENERAL setLayout(null); setSize(615, 400); //(width, height) setTitle("Formulario"); setLocationRelativeTo(null); setVisible(true); setResizable(false); setDefaultCloseOperation(EXIT_ON_CLOSE); } // JLabel que agrega una IMAGEN private JLabel Imagen1() { // Se crea el objeto JLabel JLabel imagen = new JLabel(new ImageIcon("Libreria.png")); // Le damos una ubicación dentro del Frame imagen.setBounds(470, 40, 150, 100); //(x, y, width, height) return imagen; } // Metodo Leer public void Leer()throws IOException { Scanner fileIn; String line; try{ fileIn = new Scanner(new FileReader("ArchRegistros.txt")); // csv, pdf, doc, ppt, txt, bat etc,. while(fileIn.hasNextLine()){ line = fileIn.nextLine(); JOptionPane.showMessageDialog(null,line + "n"); } fileIn.close(); } catch(FileNotFoundException e) { JOptionPane.showMessageDialog(null,"Error : " + e.getMessage()); } } // Metodo Guardar public void Guardar()throws IOException { try { PrintWriter fileOut; fileOut = new PrintWriter(new FileWriter("ArchRegistros.txt",true)); // csv, pdf, doc, ppt, txt, bat etc,. String line = ("Titulo del libro: "+tituloL.getText()+""+" Edicion: "+edicion.getText()+""+" Precio: "+""+precio.getText()+""+" Clave: "+""+clave.getText()); fileOut.println(line); setBackground(new Color(0x660000)); fileOut.close(); } catch(IOException e) { JOptionPane.showMessageDialog(null,"Error : " + e.getMessage()); } } public void actionPerformed (ActionEvent e) { if (e.getSource().equals(submenu1)) { System.exit(0); } if (e.getSource().equals(submenu3)) { try { Leer(); } catch (IOException e1) { e1.printStackTrace(); } } if (e.getSource().equals(mostrar)) { try { Leer(); } catch (IOException e1) { e1.printStackTrace(); } } if (e.getSource().equals(submenu2)) { tituloL.setText(""); edicion.setText(""); precio.setText(""); clave.setText(""); } if(e.getSource().equals(salir)) { System.exit(0); } if(e.getSource().equals(limpiar)) { tituloL.setText(""); edicion.setText(""); precio.setText(""); clave.setText(""); } if(e.getSource().equals(guardar)) { try { Guardar(); } catch (IOException e1) { e1.printStackTrace(); } } } //Main PRINCIPAL public static void main(String[] args) { //Objeto Libreria x = new Libreria(); } }
package registro; //@author Oliver //http://blog2000.blogspot.mx/ import java.io.*; import javax.swing.*; import registro.Autor; import registro.Fecha; import registro.Libro; public class Registro { public static void main(String[] args)throws IOException { //Declaracion de variables BufferedReader in = new BufferedReader(new InputStreamReader(System.in)); Autor autor1[] = new Autor[2]; Libro libro1[] = new Libro[6]; Fecha fecha1[] = new Fecha[3]; int opc,x=0,y=0,z=0; int i,j,k; //Menu do{ //Pequeño menu de seleccion opc=Integer.parseInt(JOptionPane.showInputDialog(null,"" + "<html><p style="width: 600px; background-color: #CCCCCC; border-left: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 30px;"><b><img src='file:autor.png'/>1.Ingresar datos del autor.n" + "<html><p style="width: 600px; background-color: #CCCCCC; border-left: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 30px;"><b><img src='file:libro.png'/>2.Ingresar datos del libro.n" + "<html><p style="width: 600px; background-color: #CCCCCC; border-left: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 30px;"><b><img src='file:fecha.png'/>3.Ingresar fecha.n" + "<html><p style="width: 600px; background-color: #CCCCCC; border-left: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 30px;"><b><img src='file:visualizar.png'/>4.Vizualisar.n" + "<html><p style="width: 600px; background-color: #CCCCCC; border-left: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 30px;"><b><img src='file:salir.png'/>5.Salir. ","ELIGE UNA OPCION",JOptionPane.PLAIN_MESSAGE)); switch(opc){ case 1: //Crea un objeto de tipo autor autor1[x]= new Autor(); autor1[x].AsignarAutor(); x++; break; case 2: //Crea un objeto de tipo libro libro1[y] = new Libro(); libro1[y].AsignarLibro(); y++; break; case 3: //Crea un objeto de tipo fecha fecha1[z] = new Fecha(); fecha1[z].Asignarfecha(); z++; break; case 4: //Metodo para vizualisar for(i = 0;i < x;i++){ for(j = 0;j < y;j++){ for(k = 0;k < z;k++){ Icon icono1 = new ImageIcon("information.png"); JOptionPane.showMessageDialog(null,"<html><p style="background-color: #CCCCCC; width: 400px; padding: 10px; font-family: consolas; font-size: 20px;"><b>Titulo: "+libro1[j].getTitulo()+"n<html><p style="background-color: #CCCCCC; width: 400px; padding: 10px; font-family: consolas; font-size: 20px;"><b>Edicion: "+libro1[j].getEdicion()+"n<html><p style="background-color: #CCCCCC; width: 400px; padding: 10px; font-family: consolas; font-size: 20px;"><b>Autor: "+autor1[i].getNombre()+" "+autor1[i].getApellido()+"n<html><p style="background-color: #CCCCCC; width: 400px; padding: 10px; font-family: consolas; font-size: 20px;"><b>ISBN: "+libro1[j].getISBN()+"n<html><p style="background-color: #CCCCCC; width: 400px; padding: 10px; font-family: consolas; font-size: 20px;"><b>Lugar: "+libro1[j].getLugar()+"n<html><p style="background-color: #CCCCCC; width: 400px; padding: 10px; font-family: consolas; font-size: 20px;"><b>Fecha: "+fecha1[k].getDia()+" de "+fecha1[k].getMes()+" del "+fecha1[k].getAño()+"n<html><p style="background-color: #CCCCCC; width: 400px; padding: 10px; font-family: consolas; font-size: 20px;"><b>Numero de paginas: "+libro1[j].getPaginas()+" paginas.","SALIDA",JOptionPane.INFORMATION_MESSAGE,icono1); } } } break; case 5: Icon icono2 = new ImageIcon("C:\Users\Ortuño\Documents\NetBeansProjects\Registro\information.png"); JOptionPane.showMessageDialog(null,"<html><p style="background-color: #CCCCCC; padding: 10px; font-family: consolas; font-size: 20px;"><b>Hasta pronto !","SALIDA",JOptionPane.INFORMATION_MESSAGE,icono2); break; default: Icon icono3 = new ImageIcon("C:\Users\Ortuño\Documents\NetBeansProjects\Registro\error.png"); JOptionPane.showMessageDialog(null,"<html><p style="background-color: #CCCCCC; padding: 10px; font-family: consolas; font-size: 20px;"><b>Opcion incorrecta !","MENSAJE",JOptionPane.ERROR_MESSAGE,icono3); break; } }while(opc !=5); } }
package registro; //@author Oliver //http://blog2000.blogspot.mx/ import java.io.*; import javax.swing.*; public class Autor { String nombre,apellido; public void AsignarAutor()throws IOException{ BufferedReader in = new BufferedReader(new InputStreamReader(System.in)); nombre = JOptionPane.showInputDialog(null,"<html><p style="background-color: #CCCCCC; border-left: solid 20px #FF0000; border-right: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 20px;"><b>Introduce el nombre del autor:","AUTOR",JOptionPane.PLAIN_MESSAGE); apellido = JOptionPane.showInputDialog(null,"<html><p style="background-color: #CCCCCC; border-left: solid 20px #FF0000; border-right: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 20px;"><b>Introduce el apellido del autor:","AUTOR",JOptionPane.PLAIN_MESSAGE); } public String getApellido() { return apellido; } public void setApellido(String apellido) { this.apellido = apellido; } public String getNombre() { return nombre; } public void setNombre(String nombre) { this.nombre = nombre; } }
package registro; //@author Oliver //http://blog2000.blogspot.mx/ import java.io.*; import javax.swing.*; public class Libro { String titulo; String ISBN; String paginas; String edicion; String editorial; String lugar; public void AsignarLibro()throws IOException{ BufferedReader in = new BufferedReader(new InputStreamReader(System.in)); titulo = JOptionPane.showInputDialog(null,"<html><p style="background-color: #CCCCCC; border-left: solid 20px #FF0000; border-right: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 20px;"><b>Introduce el titulo:","LIBRO",JOptionPane.PLAIN_MESSAGE); ISBN = JOptionPane.showInputDialog(null,"<html><p style="background-color: #CCCCCC; border-left: solid 20px #FF0000; border-right: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 20px;"><b>Introduce el ISBN:","LIBRO",JOptionPane.PLAIN_MESSAGE); paginas = JOptionPane.showInputDialog(null,"<html><p style="background-color: #CCCCCC; border-left: solid 20px #FF0000; border-right: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 20px;"><b>Introduce el numero de paginas:","LIBRO",JOptionPane.PLAIN_MESSAGE); edicion = JOptionPane.showInputDialog(null,"<html><p style="background-color: #CCCCCC; border-left: solid 20px #FF0000; border-right: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 20px;"><b>Introduce la edicion:","LIBRO",JOptionPane.PLAIN_MESSAGE); editorial = JOptionPane.showInputDialog(null,"<html><p style="background-color: #CCCCCC; border-left: solid 20px #FF0000; border-right: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 20px;"><b>Introduce la editorial:","LIBRO",JOptionPane.PLAIN_MESSAGE); lugar = JOptionPane.showInputDialog(null,"<html><p style="background-color: #CCCCCC; border-left: solid 20px #FF0000; border-right: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 20px;"><b>Introduce el lugar:","LIBRO",JOptionPane.PLAIN_MESSAGE); } public String getISBN() { return ISBN; } public void setISBN(String ISBN) { this.ISBN = ISBN; } public String getEdicion() { return edicion; } public void setEdicion(String edicion) { this.edicion = edicion; } public String getEditorial() { return editorial; } public void setEditorial(String editorial) { this.editorial = editorial; } public String getLugar() { return lugar; } public void setLugar(String lugar) { this.lugar = lugar; } public String getPaginas() { return paginas; } public void setPaginas(String paginas) { this.paginas = paginas; } public String getTitulo() { return titulo; } public void setTitulo(String titulo) { this.titulo = titulo; } }
package registro; //@author Oliver //http://blog2000.blogspot.mx/ import java.io.*; import javax.swing.*; public class Fecha { String dia,mes,año; public void Asignarfecha()throws IOException{ BufferedReader in = new BufferedReader(new InputStreamReader(System.in)); dia = JOptionPane.showInputDialog(null,"<html><p style="background-color: #CCCCCC; border-left: solid 20px #FF0000; border-right: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 20px;"><b>Introduce el dia:","FECHA",JOptionPane.PLAIN_MESSAGE); mes = JOptionPane.showInputDialog(null,"<html><p style="background-color: #CCCCCC; border-left: solid 20px #FF0000; border-right: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 20px;"><b>Introduce el mes:","FECHA",JOptionPane.PLAIN_MESSAGE); año = JOptionPane.showInputDialog(null,"<html><p style="background-color: #CCCCCC; border-left: solid 20px #FF0000; border-right: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 20px;"><b>Introduce el año:","FECHA",JOptionPane.PLAIN_MESSAGE); } public String getAño() { return año; } public void setAño(String año) { this.año = año; } public String getDia() { return dia; } public void setDia(String dia) { this.dia = dia; } public String getMes() { return mes; } public void setMes(String mes) { this.mes = mes; } }
package dlibros; //@author Oliver //http://blog2000.blogspot.mx/ import java.io.*; import java.awt.*; import javax.swing.*; public class DLibros { // Objeto agenda static Datos[]obj=new Datos[3]; //Menu para elegir una opcion public static int menu(){ String opt=JOptionPane.showInputDialog(null,"" + "<html><p style="width: 500px; background-color: #CCCCCC; border-left: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 30px;"><b><img src='file:insert1.png'/>1. Insertar registro" + "n<html><p style="width: 500px; background-color: #CCCCCC; border-left: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 30px;"><b><img src='file:list1.png'/>2. Mostrar registro" + "n<html><p style="width: 500px; background-color: #CCCCCC; border-left: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 30px;"><b><img src='file:search1.png'/>3. Buscar un registro" + "n<html><p style="width: 500px; background-color: #CCCCCC; border-left: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 30px;"><b><img src='file:leave1.png'/>4. Salirn","ELIGE UNA OPCION",JOptionPane.PLAIN_MESSAGE); int op=Integer.parseInt(opt); return op; } //Main principal public static void main(String[] args) throws FileNotFoundException, IOException,ClassNotFoundException{ int x=0; do{ x=menu(); switch(x){ case 1: //Captura datos try{ for(int i=0;i<3;i++){ obj[i]=new Datos(); obj[i].captura(); } FileOutputStream a=new FileOutputStream("Registro.txt"); ObjectOutputStream aa=new ObjectOutputStream(a); aa.writeObject(obj); aa.flush(); aa.close(); } catch(IOException e){ Icon icono = new ImageIcon("C:\Users\Ortuño\Documents\NetBeansProjects\Dialogos\error.png"); JOptionPane.showMessageDialog(null,"Error al escribir !","MENSAJE",JOptionPane.ERROR_MESSAGE,icono); } break; case 2: // Abrir el archivo (Imprimir) FileInputStream b=new FileInputStream("Registro.txt"); ObjectInputStream bb=new ObjectInputStream(b); obj=(Datos[])bb.readObject(); bb.close(); JTextArea Salida1= new JTextArea(); String cad1="LISTA:n"; for(int i=0;i<3;i++){ cad1+=("n"+obj[i].autor+" "+obj[i].titulo+" "+obj[i].precio+" "+obj[i].codigo+"n"); Salida1.setText(cad1); Salida1.setFont(new Font("consolas", 0, 30)); Salida1.setForeground(new Color(0x000000)); Salida1.setBackground(new Color(0xDDDDDD)); } Icon icono0 = new ImageIcon("C:\Users\Ortuño\Documents\NetBeansProjects\Dialogos\information.png"); JOptionPane.showMessageDialog(null,Salida1,"REGISTRO",JOptionPane.INFORMATION_MESSAGE,icono0); break; case 3: // Abrir el archivo FileInputStream c=new FileInputStream("Registro.txt"); ObjectInputStream cc=new ObjectInputStream(c); obj=(Datos[])cc.readObject(); cc.close(); String nombre=JOptionPane.showInputDialog(null,"<html><p style="border-left: solid 20px #0000FF; border-right: solid 20px #0000FF; padding: 10px; font-family: consolas; font-size: 20px;"><b>Nombre a buscar","BUSQUEDA",JOptionPane.PLAIN_MESSAGE); for(int i=0;i<3;i++) if(obj[i].autor.equalsIgnoreCase(nombre)){ JTextArea Salida2= new JTextArea(); String cad2=""; cad2+=("nAUTOR:"+obj[i].autor+" TITULO:"+obj[i].titulo+" PRECIO:"+obj[i].precio+" CODIGO:"+obj[i].codigo+"n"); Salida2.setText(cad2); Salida2.setFont(new Font("consolas", 0, 30)); Salida2.setForeground(new Color(0x000000)); Salida2.setBackground(new Color(0xDDDDDD)); Icon icono1 = new ImageIcon("information.png"); JOptionPane.showMessageDialog(null,Salida2,"RESULTADOS DE BUSQUEDA",JOptionPane.INFORMATION_MESSAGE,icono1); } break; case 4: Icon icono2 = new ImageIcon("information.png"); JOptionPane.showMessageDialog(null,"<html><p style="padding: 10px; font-family: consolas; font-size: 20px;"><b>Hasta luego !","SALIDA",JOptionPane.INFORMATION_MESSAGE,icono2); break; default: Icon icono3 = new ImageIcon("error.png"); JOptionPane.showMessageDialog(null,"<html><p style="padding: 10px; font-family: consolas; font-size: 20px;"><b>Opcion incorrecta !","MENSAJE",JOptionPane.ERROR_MESSAGE,icono3); break; } } while(x==1|x==2|x==3|x!=4); } }
package dlibros; //@author Oliver //http://blog2000.blogspot.mx/ import java.io.*; import javax.swing.*; public class Datos implements Serializable{ String autor, titulo, precio, codigo; public void captura(){ autor=JOptionPane.showInputDialog(null,"<html><p style="border-left: solid 20px #0000FF; border-right: solid 20px #0000FF; padding: 10px; font-family: consolas; font-size: 20px;"><b>Nombre del autor","AUTOR",JOptionPane.PLAIN_MESSAGE); titulo=JOptionPane.showInputDialog(null,"<html><p style="border-left: solid 20px #0000FF; border-right: solid 20px #0000FF; padding: 10px; font-family: consolas; font-size: 20px;"><b>Introduce el titulo","TITULO",JOptionPane.PLAIN_MESSAGE); precio=JOptionPane.showInputDialog(null,"<html><p style="border-left: solid 20px #0000FF; border-right: solid 20px #0000FF; padding: 10px; font-family: consolas; font-size: 20px;"><b>Introduce el precio","PRECIO",JOptionPane.PLAIN_MESSAGE); codigo=JOptionPane.showInputDialog(null,"<html><p style="border-left: solid 20px #0000FF; border-right: solid 20px #0000FF; padding: 10px; font-family: consolas; font-size: 20px;"><b>Introduce el codigo","CODIGO",JOptionPane.PLAIN_MESSAGE); } }
https://blogger.googleusercontent.com/img/b/R29vZ2xl/AVvXsEiDuve5518gOqa9gJl_Xfnv-7bv5WVVBsurIhLpfVYN6H28ZX_8iHHvjtViMwbkQrx62kErrpSKcsVUHXhI5fENlpAkj0R0CtR5IxjS9rUMRSSgqUXuq1u1bFwRn-2Upmh6iR5RvYnlJBs/220-h/User.png
https://blogger.googleusercontent.com/img/b/R29vZ2xl/AVvXsEiDuve5518gOqa9gJl_Xfnv-7bv5WVVBsurIhLpfVYN6H28ZX_8iHHvjtViMwbkQrx62kErrpSKcsVUHXhI5fENlpAkj0R0CtR5IxjS9rUMRSSgqUXuq1u1bFwRn-2Upmh6iR5RvYnlJBs/1600-h/User.png
https://lh5.googleusercontent.com/-VbZkpqPDOKU/AAAAAAAAAAI/AAAAAAAAAB4/PsQhM5eYaCg/s220-c/photo.jpg
https://lh5.googleusercontent.com/-VbZkpqPDOKU/AAAAAAAAAAI/AAAAAAAAAB4/PsQhM5eYaCg/s1600-c/photo.jpg
https://yt3.ggpht.com/-IKNCdmKeh_M/AAAAAAAAAAI/AAAAAAAAAAA/MECOkqpQWB0/s100-c-k-no/photo.jpg
https://yt3.ggpht.com/-IKNCdmKeh_M/AAAAAAAAAAI/AAAAAAAAAAA/MECOkqpQWB0/s1600-c-k-no/photo.jpg
// Librería para mensajes de dialogo import javax.swing.JOptionPane; // Clase dialogo public class Dialogo { // Main principal public static void main (String[] args) { // Variable tipo String String nombre; // Método con mensaje de entrada nombre = JOptionPane.showInputDialog(null,"Ingresa tu nombre: "); // Método con mensaje de salida JOptionPane.showMessageDialog(null,"Tu nombre es: "+nombre); } }
// Método con mensaje de entrada nombre = JOptionPane.showInputDialog(null,"Ingresa tu nombre: ","Titulo",JOptionPane.PLAIN_MESSAGE); // Método con mensaje de salida JOptionPane.showMessageDialog(null,"Tu nombre es: "+nombre,"Resultado",JOptionPane.PLAIN_MESSAGE);
Ejemplo de mensaje | Código |
---|---|
![]() | JOptionPane.PLAIN_MESSAGE |
![]() | JOptionPane.INFORMATION_MESSAGE |
![]() | JOptionPane.QUESTION_MESSAGE |
![]() | JOptionPane.WARNING_MESSAGE |
![]() | JOptionPane.ERROR_MESSAGE |
// Librería para todas sus componentes import javax.swing.*; // Clase dialogo public class Dialogo { // Main principal public static void main (String[] args) { // Objeto icono Icon icono = new ImageIcon("C:\\Usuario\\Administrador\\Escritorio\\Iconos\\Bicho.png"); // Método con mensaje de salida JOptionPane.showMessageDialog(null,"MENSAJE QUE DESEES","Titulo de mensaje",JOptionPane.INFORMATION_MESSAGE,icono); } }
Recibe en tu correo las últimas noticias del blog. Sólo ingresa tu correo para suscribirte.